Implementing Cover Trades
The Cover Trades feature, available from version 2.32 of FX Sales, provides an alternative means of trading to the standard RFS ticket. In a time-critical sales opportunity, the sales dealer can agree a price with a client and use Cover Trades to execute a trade immediately at a zero-margin rate. After execution, the dealer amends the margin on the trade so that the all-in rate matches the price quoted to the client.
This page describes how to implement Cover Trades in your integration adapter.
Feature overview
When an FX Sales user selects a TOBO user for whom Cover Trades is enabled, the Pending tile set is shown in the UI:
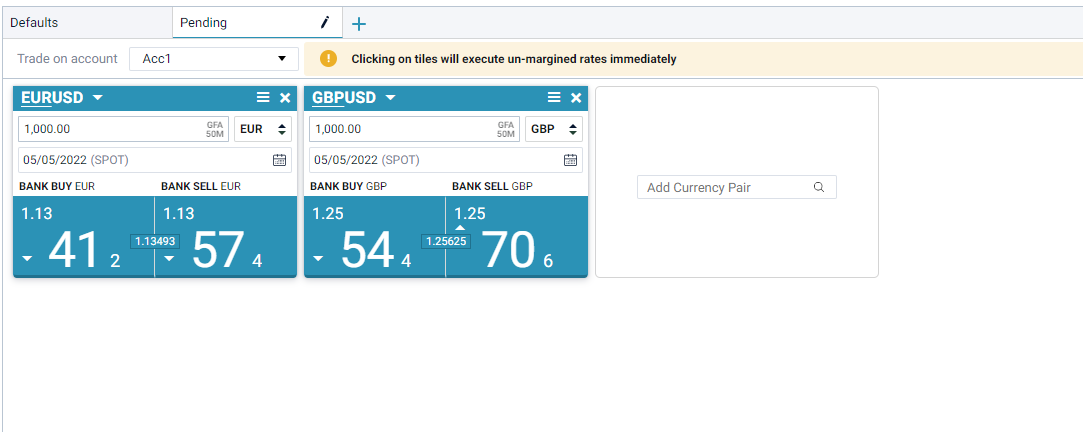
Cover Trade tiles display executable, zero-margin rates. You can customise how many mouse clicks are required to execute a trade (single-click, double-click, or single-click with confirmation dialog). See Implement execution of Cover Trades.
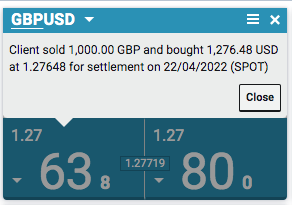
Following execution of a cover trade, the trade is available in the blotter for immediate amendment. The sales dealer amends the margin so that the all-in rate matches the price agreed with the client:
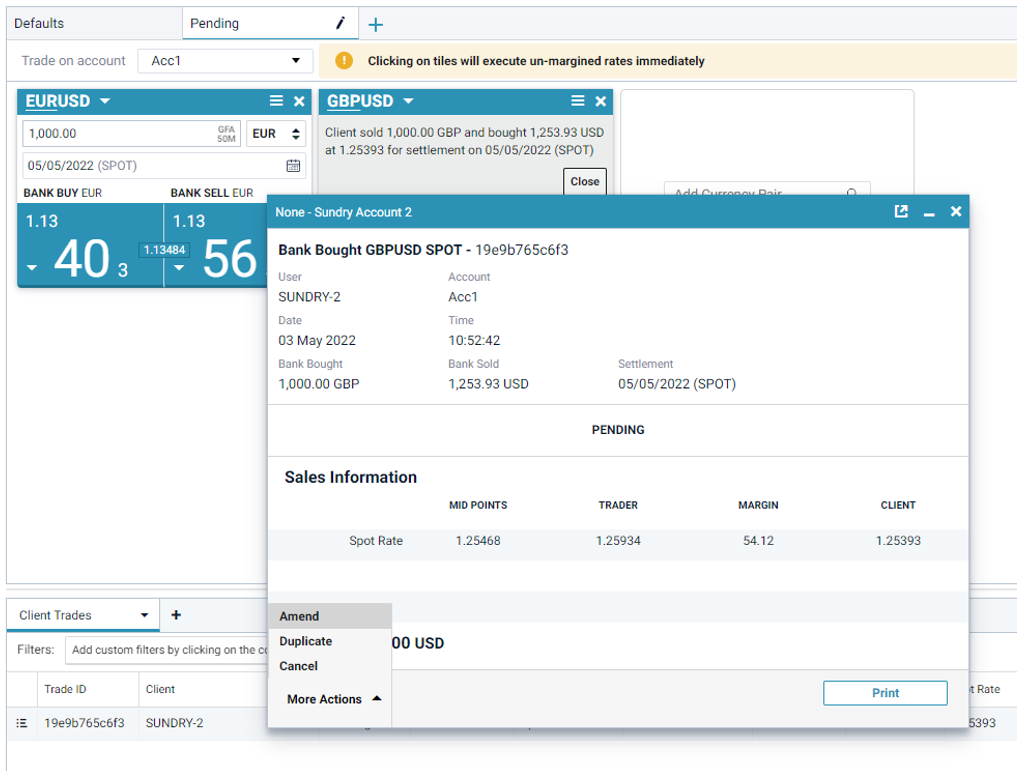
Implementation requirements
To implement FX Sales Cover Trades, you require the following:
-
FX Sales 2.32
-
Integration adapters implementing the following FX Integration API (3.68.0+) features:
The implementations of the User Config Service, ESP Trade Model, and the Amend Trade Model can be in the same or separate adapters.
Implementation overview
Implementation requires three steps:
-
User configuration: update your User Config Service implementation to enable the Cover Trades feature for TOBO users.
-
Pricing: update your trading adapter’s ESP pricing subscription handler to supply zero-margin rates for Cover Trade pricing subscriptions.
-
Trading: update your trading adapter’s ESP trade model Submit handler
Implement user configuration for Cover Trades
In your User Config Adapter’s implementation of CachedObjectProvider<TOBOConfig, SalesTOBOConfigSubjectInfo>.onRequest
, perform the following steps:
-
Refer to your backend customer profile to determine if the TOBO user represented by the
SalesTOBOConfigSubjectInfo
parameter should have Cover Trades enabled. -
If Cover Trades should be enabled for the TOBO user, register an enabled
CoverTrade
object with theFeatures
object:
public class ToboConfigProvider implements
CachedObjectProvider<TOBOConfig, SalesTOBOConfigSubjectInfo> {
@Override
public void onRequest(
final SalesTOBOConfigSubjectInfo salesToboConfigSubjectInfo,
final SubjectConsumer<TOBOConfig> objectPublisherConsumer) {
...
Features features = new Features();
features.coverTrade(new CoverTrade().enabled(true));
...
ToboConfig toboConfig = new ToboConfig();
toboConfig.setFeatures(features);
objectPublisherConsumer.accept(toboConfig);
}
...
}
Implement pricing for Cover Trades
In your trading adapter, use the RateSubjectInfo.isCoverTrade()
) method to identify the margin requirements of a pricing subscription.
When RateSubjectInfo.isCoverTrade()
returns true, your trading adapter should provide rates with no TOBO user margin applied:
Implement execution of Cover Trades
Sales dealers execute a cover trade by clicking a rate in a Cover Trade tile. To configure how many mouse clicks are required to execute a trade, set the AppConfig option FX_TILE_ESP_TRADING_CLICK_TYPE
to one of the strings below:
-
ONE_CLICK
: single click -
DOUBLE_CLICK
: double click -
TWO_CLICK
: single click with a confirmation dialog (OK/Cancel)
When your trading adapter receives an ESP Submit message from a Cover Trade tile, the message includes the field IsCoverTrade
. This field is retrievable in your trading adapter using the SubmitTradeEvent.getIsCoverTrade() method.
Handle cover trades as you would any other ESP trade, with one exception: add the IsAmendable
field to the execution blotter record. The TradeConfirmation event for a cover trade does not require any extra fields.
This is the minimum that is required to implement Cover Trades. You may wish to extend this implementation to make it easier to identify unamended cover trades in the execution blotter. For example, in addition to adding the IsAmendable
field to the Execution Blotter record, you could also set a custom status, such as "Pending".